What is an API
An API (Application Programming Interface) is a mechanism through which two pieces of software communicate.
If you were like me a few years ago, you might wonder, "What exactly does that mean?"
Let's look at an analogy.
Imagine an automatic coffee machine. The machine has buttons on the front (espresso, cappuccino, hot water, etc.). Additionally, the machine has components and software that prepare the coffee.
The API is like the buttons of the coffe machine. The buttons on the machine define how you can communicate with a system you don’t know its inner workings. Thus, every piece of software has an API (like the buttons on the coffee machine) that allows it to communicate with other software components.
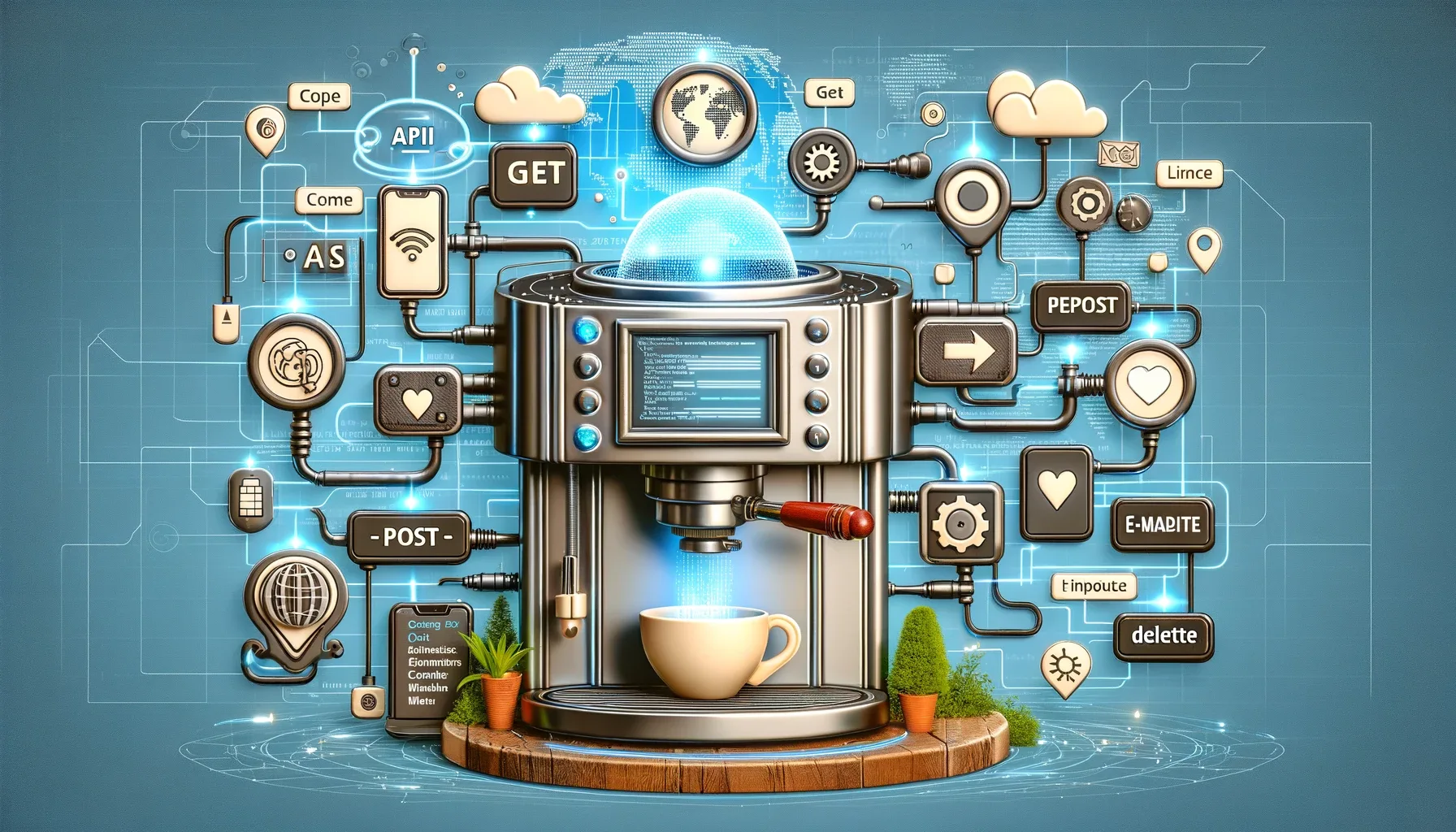
Real-Life Examples of APIs
Before I explain why developers create APIs, let's look at some real-life examples to understand better how they function:
Social Media: The Twitter API, for example, allows developers to retrieve tweets, send tweets, or delete tweets through their applications. This enables the creation of specialized tools that leverage Twitter data.
E-commerce: Websites like Skroutz provide APIs to communicate with e-shops. Thus, each store participating in the Skroutz marketplace can update Skroutz about changes in inventory, products, and prices.
Electronic Maps: APIs like those offered by Google Maps allow developers to integrate maps, routes, and geographic data into their applications. Any application that uses maps doesn't build them from scratch; it uses ready-made APIs.
Weather Services: Services like OpenWeatherMap offer APIs that allow developers to obtain current and forecasted weather information. For example, your running app can notify you that it will rain at 7 PM and ask if you want to reschedule your workout.
Benefits of APIs
Now that you understand what an API is, let's see why they are useful.
Modularity: APIs allow developers to create applications combining distinct software components. Thus, different functions of an application can be developed independently and communicate effectively. Each unit can be updated or fixed without affecting the rest of the system.
Reusability: Functions can be easily reused through APIs. Once an API is developed for a specific service, it can be repeatedly used in different parts of an application or even in different projects. This saves time and resources and ensures consistency in the implementation of functions.
Simplification: APIs simplify the development of applications by hiding the operation of the backend from the frontend. Developers can use APIs to interact with a server or a database without knowing the implementation details.
Integration: By providing a common protocol, APIs facilitate communication between software systems. Thus, we can quickly and directly connect with third-party software. For example, we can integrate payments into our application using a bank's API.
Security: APIs enforce strict authentication, encryption, and data validation processes, enhancing the security of our software.
Scalability: APIs divide the software into individual units. Thus, each unit can be maintained and expanded easier.
How an API is Defined : Endpoints + Documentation
The fundamental parts that define an API are the endpoints and the documentation.
Endpoints: These are specific URLs provided by the API to perform different functions (like the various buttons on a coffee machine). For example, an endpoint in a social media API might allow you to retrieve a user's friend list and could look something like this:
https://someservice/api/users/userId/friend-list
Documentation: These documents describe how to use the endpoints (like the instructions for the coffee machine). Here, you'll find the parameters you need to send, what endpoints exist, the data they provide, and possible issues you might encounter.
Types of APIs
Based on their use and accessibility, APIs are distinguished into the following categories:
Data APIs | Connect applications to database management systems |
Operating system APIs | Define how applications use the services and resources of the operating system |
Remote APIs | Define how applications communicate across different devices |
Web APIs | Allow the transfer of data and functionality over the internet |
Web APIs are the most popular because they enable access to applications and data from anywhere in the world.
Type of Web APIs
Open / Public APIs | APIs available to everyone |
Partner APIs | Connect business partners and require authentication. |
Internal / Private APIs | APIs that are used only within a single company or organization |
Composite APIs | Allow developers to access multiple endpoints with a single call |
What is a REST API?
REST (Representational State Transfer) is a standard for developing web applications. REST APIs use HTTP requests to exchange data. They allow for data retrieval, creation, modification, and deletion through well-defined methods such as GET, POST, PUT, and DELETE.
REST APIs are stateless, meaning there is no correlation between different calls to the API. Each call is independent. Due to their simplicity, REST APIs are the most popular pattern for developing modern web and mobile applications.
How to Use an API
To use an API, you should do the following:
Understanding the API: Read the API's documentation for its functions and endpoints.
Authentication: APIs often require access keys to be used. Check the documentation on how to obtain one.
Making Requests: Call the API via HTTP. You can do this using any programming language, a terminal, or a tool like Postman. The most common languages for calling an API are Python, JavaScript, or Java.
Example: Using an API
Here is an example in Javascript to retrieve data from the JSONPlaceholder API:
// We write the URL for the endpoint that returns a todo
const url = "https://jsonplaceholder.typicode.com/todos/1";
// We call the API to get the todo
fetch(url)
.then(response => response.json())
.then(json => console.log(json))
You can run the above code in an online JavaScript compiler like this one.
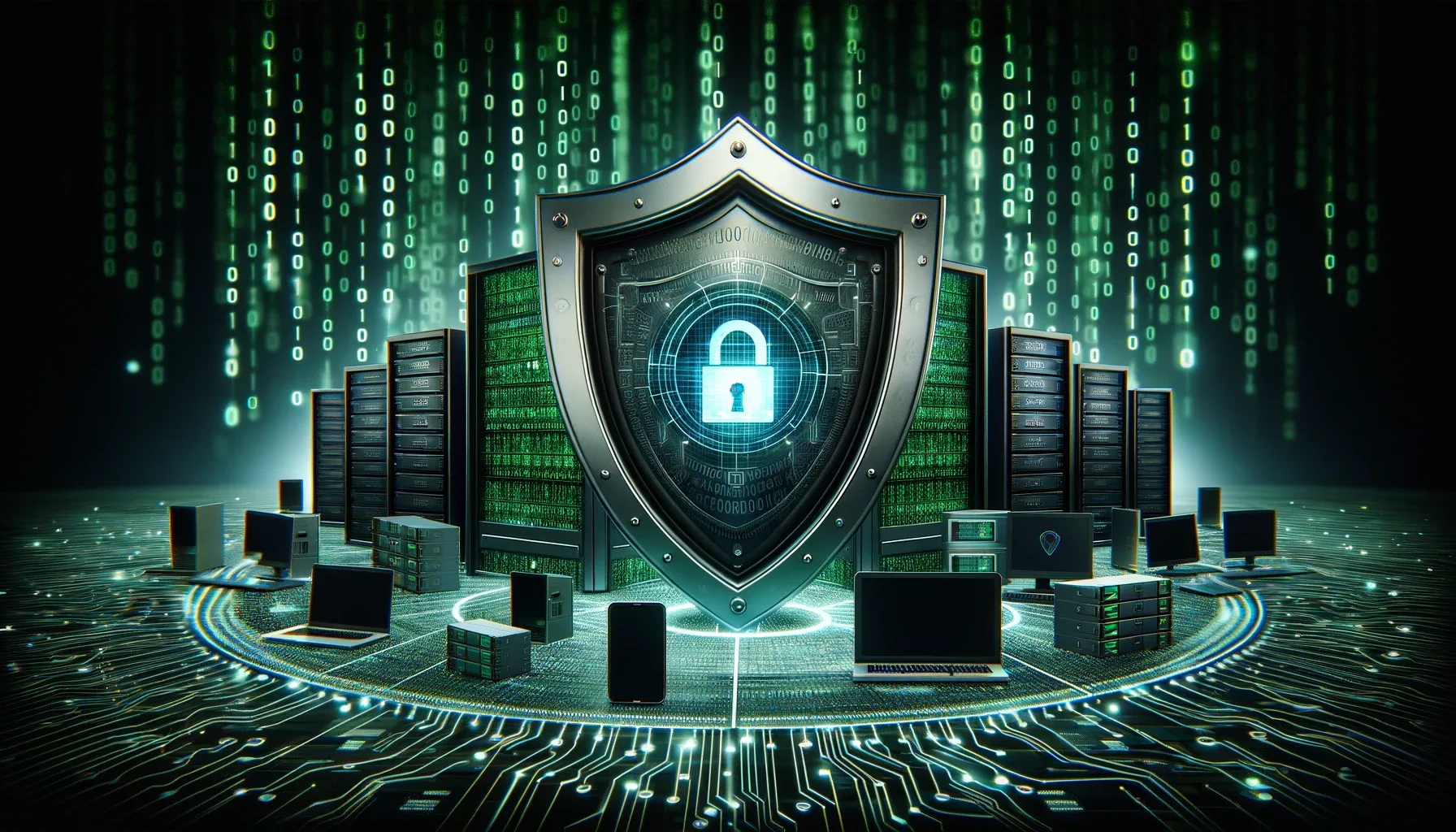
API Security
Security is critically important when using or developing APIs, as sensitive data and functions must be protected from the public. Key ways to secure your API include:
HTTPS: Use HTTPS instead of HTTP for your API to ensure data encryption during transmission.
Authentication and Authorization: Use mechanisms such as OAuth and JWT tokens to control who can access the API.
Rate Limiting: Limit the number of calls a user can make within a certain period to prevent DoS attacks.