Certainly, you've heard the term "algorithm." The word "algorithm" is often associated with an abstract concept, as something complex or related to mathematics. However, in reality, it's much simpler.
What is an Algorithm
To define an algorithm, you need a starting and an ending state.
An algorithm is a series of commands that take you from a starting to an ending state.
The starting state is also called the input of the algorithm. The ending state is called the output of the algorithm.
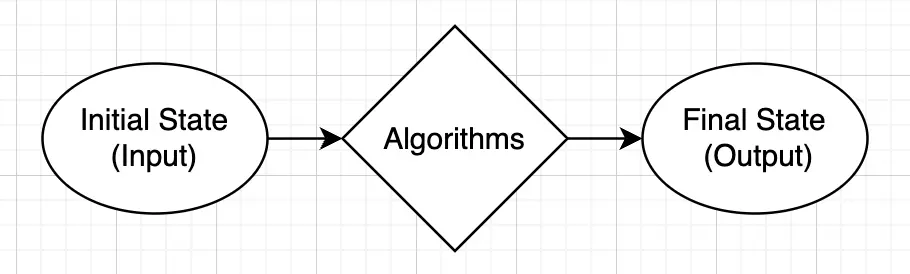
Algorithm Example
Imagine you wake up in the morning and want to drink orange juice. Starting state (Input): You want to drink orange juice. Ending state (Output): You have a glass of orange juice in your hands.
Algorithm:
Material preparation:
Find two or more ripe oranges.
You have a juicer or an electric orange juicer.
Prepare a glass or container to collect the juice
Preparing the oranges:
Cut the oranges in half horizontally (from the stem to the base).
Juicing:
Take half of an orange and place it on the juicer.
Apply pressure by pressing and turning the orange on the juicer to extract as much juice as possible.
Repeat the process for the other half of the orange and for all the oranges you have.
Juice collection:
Pour the juice from the juicer container into your glass.
Thus, the algorithm is the process that leads you from the initial state (oranges) to the final state (orange juice).
Why We Need Algorithms
Algorithms are useful because they offer solutions to problems. Whether you want to make a dessert, tie your shoelaces, or sort numbers from smallest to largest, you need an algorithm.
Once someone creates an algorithm, they can share the solution with you. This way, not everyone has to solve the same problem.
This is particularly useful in computer science, where algorithms play the most crucial role. For example, computing systems use an algorithm that efficiently sorts numbers billions of times daily.
What is a Good Algorithm
For an algorithm to be good, it must do the following:
Correctly solve the problem.
It must give us the correct result (output) for every possible input.
Be efficient.
In programming, an algorithm's efficiency is critical. It’s measured in terms of time complexity (how the execution time changes as the input size changes) and space complexity (how much memory it uses).
Be scalable.
Meaning it can work efficiently and quickly for both small and large inputs. For example, a sorting algorithm works quickly and correctly for 2 and 2.000.000 million numbers.
Algorithms You Use Daily
Dijkstra:
This algorithm finds the shortest of all possible paths to go from point A to point B. It is used by the navigation systems you use every day.
Cryptography Algorithms:
It is used to keep your data safe, whether we're talking about your conversations on social media or your e-banking.
PageRank:
This is the algorithm Google uses to rank websites in your search results.
Recommendation Algorithms:
Analyze your behavior and preferences to make suggestions. Netflix uses them to suggest series to you, Spotify to suggest songs, and e-shops to suggest related products.
Facial Recognition Algorithms:
Allows you to unlock your mobile phone with your face (FaceID).
Social Media Algorithms:
Used by social networking platforms to decide what you'll see on your homepage.
Weather Forecasting Algorithms:
Analyze historical data and current weather conditions to find patterns and accurately predict the weather for the coming period.
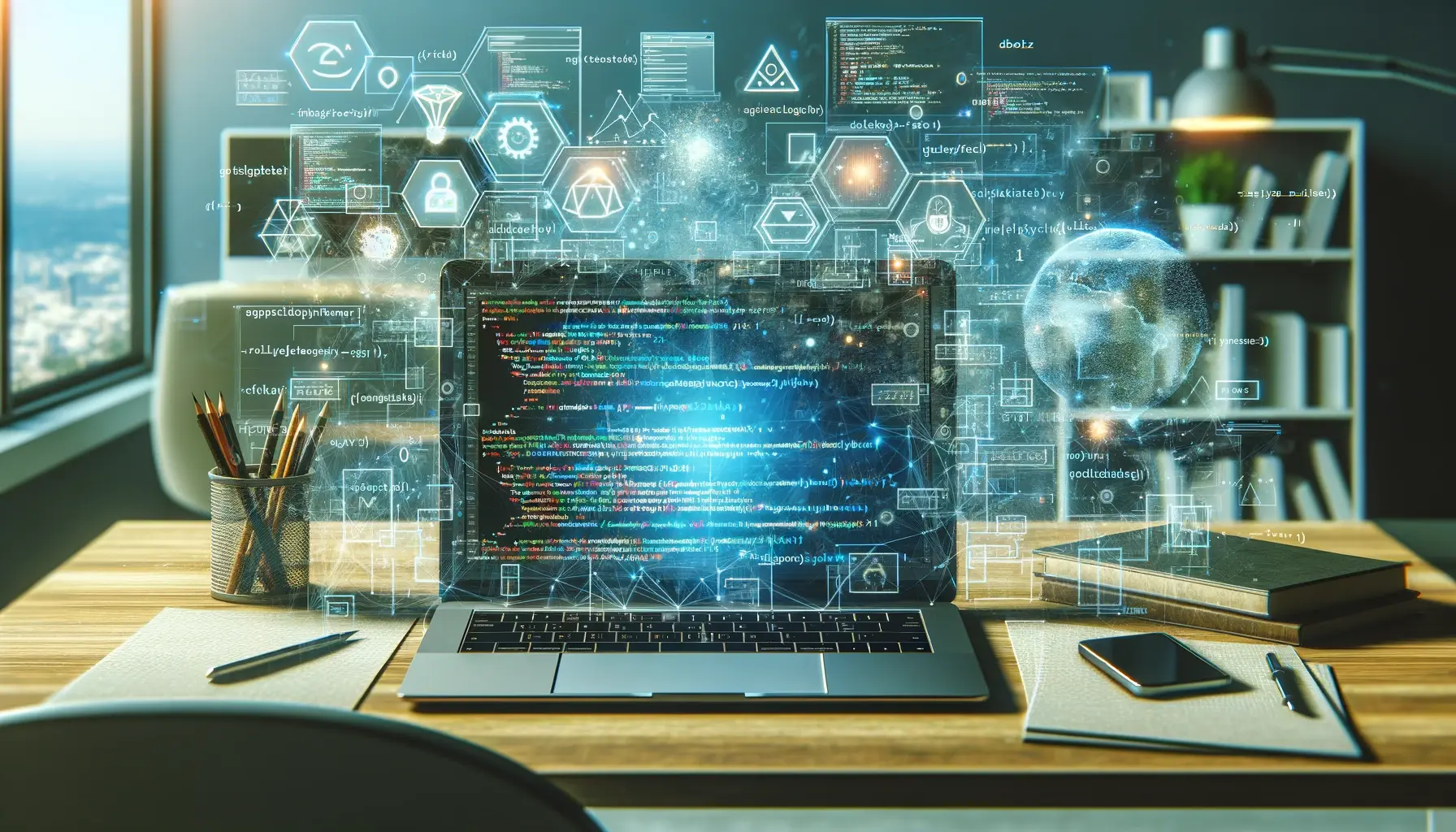
What is an Algorithm in Programming
In programming, an algorithm is a precise set of instructions designed to lead us from a starting to an ending state. The definition is the same as the one we discussed above. The only difference is that in programming, algorithms are executed by computers.
The algorithm can range from simple data calculations to complex problem-solving operations.
All programming algorithms must be written in Programming Languages. Algorithms must be expressed in a language that computers can interpret, such as Python, Java, or C++. Each language has its syntax and style, but the core logic of the algorithm remains constant across languages.
Algorithms are the building blocks of software. Whether a social media platform sorts posts by relevance or a navigation app calculates the quickest route, algorithms drive the functionality.
Algorithm Design Techniques
Name | Method | Example |
Brute Force | Tries all possible solutions | Password Cracking |
Recursion | Converts the problem to recursive relation | Calculating factorial |
Hashing | Utilizes hash functions | Quick lookup |
Divide and Conquer | Divides a problem into sub-problems | Sorting numbers |
Greedy Algorithms | Makes the optimal choice at each step. | Finding Shortest path |
Dynamic Programming | Saves repeating calculations | Fibonacci sequence |
Randomness | Uses chance | Monte Carlo methods |
Brute Force: The algorithm tries all possible solutions until it finds the right one. It's easy to think about and understand. The more possible solutions there are, the slower the algorithm becomes.
Recursion: The algorithm breaks the problem into smaller parts, which it solves based on a recursive relation.
Hashing: The algorithm transforms data into a value using a hash function. This transformation allows us to find and modify data quickly. It is often used in databases.
Divide and Conquer:
This strategy divides a problem into sub-problems, solves each independently, and then combines their solutions. It’s a powerful method for tackling complex problems by simplifying them.
Greedy Algorithms:
These algorithms make the most optimal choice at each step, aiming for a local optimum in hopes of finding a global optimum. While efficient, they're not universally applicable to all problems.
Dynamic Programming:
Saves results of intermediate steps to avoid recalculating them, significantly speeding up the computation for problems with overlapping subproblems.
Randomness:
Incorporates chance into the decision-making process, which can be particularly effective when a good-enough solution is acceptable or deterministic solutions are impractical.